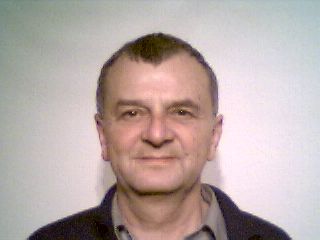 |
Course 3D_XNA: 3D-Computer Graphics with XNA Chapter C1: The Complete Code of the Moving Triangles Project
Copyright © by V. Miszalok, last update: 2011-03-14
|
This project is the XNA-clone of
3D-Computer Graphics with Managed DirectX, Chapter C1: Moving Triangles and of
3D-Computer Graphics with C++ and OpenGL, Chapter C1: Moving Triangles.
Project triangle1
This chapter requires no DirectX SDK but:
1. Visual C# 2019 Express or Visual Studio 2010 Professional
2. XNA Game Studio 4.0
3. DirectX End-User Runtimes
1. Main Menu after starting VC# 2010:
File →
New Project... →
Project types: XNA GameStudio 4.0 →
Templates: Windows Game (4.0) →
Name: triangle1 →
Location: C:\temp →
Create directory for solution: switch it off → OK.
Solution Explorer - triangle1: Delete the file Program.cs and the default code of Game1.cs.
2. If You find no Solution Explorer-window, open it via the main menu: View → Solution Explorer.
Inside the Solution Explorer-window click the plus-sign in front of triangle1. A tree opens. Look for the branch "References". Check if there are four references Microsoft.XNA.Framework, Microsoft.XNA.Framework.Game, mscorlib and System.
3. You should switch off the vexatious automatic format- and indent- mechanism of the code editor before You copy the following code to triangle1.cs (otherwise all the code will be reformatted into chaos):
3.1. Main menu of VC# 2010: click menu "Tools".
3.2. A DropDown-menu appears. Click "Options...".
3.3. An Options dialog box appears.
3.4. Double click the branch "Text Editor" → double click "C#" → double click "Formatting".
3.5. A sub-tree appears with the 5 branches "General, Indentation, New Lines, Spacing, Wrapping".
3.6. Uncheck any of the 45 check boxes that these 5 Formatting-branches may offer.
3.7. Leave the dialog box with button "OK".
4. Copy the following code into the empty Game1.cs:
using System;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
static class Program
{ static void Main() { Game1 game = new Game1(); game.Run(); }
public class Game1 : Microsoft.Xna.Framework.Game
{ private GraphicsDeviceManager g;
private VertexBuffer vbuffer;
private BasicEffect effect;
private EffectPass pass;
private float rotAngle = 0f;
private Matrix rot = Matrix.CreateRotationY( 0f );
private Matrix zoom = Matrix.CreateScale( 0.5f );
private Matrix leftshift = Matrix.CreateTranslation( new Vector3( -1f, 1f, 0f ) );
private Matrix rightshift = Matrix.CreateTranslation( new Vector3( 1f, 0f, 0f ) );
private const Int32 nTriangles = 100;
private float[] dx = new float[nTriangles];
private float[] dy = new float[nTriangles];
private float[] dz = new float[nTriangles];
private float[] ax = new float[nTriangles];
private float[] ay = new float[nTriangles];
private float[] az = new float[nTriangles];
private Random r = new Random();
public Game1()
{ Window.Title = "3DTriangleAnimation";
Window.AllowUserResizing = true;
g = new GraphicsDeviceManager( this );
g.IsFullScreen = false;
g.PreferredBackBufferWidth = 600;
g.PreferredBackBufferHeight = 600;
}
protected override void Initialize()
{ VertexPositionColor[] v = new VertexPositionColor[3];
v[0].Position.X=-1f; v[0].Position.Y=-1f; v[0].Position.Z=0f;
v[1].Position.X= 0f; v[1].Position.Y= 1f; v[1].Position.Z=0f;
v[2].Position.X= 1f; v[2].Position.Y=-1f; v[2].Position.Z=0f;
v[0].Color = Color.DarkGoldenrod;
v[1].Color = Color.Cornsilk;
v[2].Color = Color.MediumOrchid;
vbuffer = new VertexBuffer( g.GraphicsDevice, typeof(VertexPositionColor), 3, BufferUsage.WriteOnly );
vbuffer.SetData< VertexPositionColor >( v );
effect = new BasicEffect( g.GraphicsDevice, null );
effect.View = Matrix.CreateLookAt( new Vector3(0, 0, 3), Vector3.Zero, Vector3.Up );
effect.Projection = Matrix.CreatePerspectiveFieldOfView( MathHelper.Pi/4, 1f, 1f, 10f );
effect.VertexColorEnabled = true;
pass = effect.CurrentTechnique.Passes[0];
for ( int i = 0; i < nTriangles; i++ )
{ dx[i] = (float)r.NextDouble() - 0.5f; //random permanent translation dx
dy[i] = (float)r.NextDouble() - 0.5f; //random permanent translation dy
dz[i] = (float)r.NextDouble() - 0.5f; //random permanent translation dz
ax[i] = (float)r.NextDouble(); //random initial pitch rotation angle
ay[i] = (float)r.NextDouble(); //random initial yaw rotation angle
az[i] = (float)r.NextDouble(); //random initial roll rotation angle
}
//g.GraphicsDevice.RenderState.DepthBufferEnable = false;
//g.GraphicsDevice.RenderState.DepthBufferWriteEnable = false;
}
protected override void Update( GameTime gameTime )
{ g.GraphicsDevice.Vertices[0].SetSource( vbuffer, 0, VertexPositionColor.SizeInBytes );
g.GraphicsDevice.VertexDeclaration = new VertexDeclaration( g.GraphicsDevice, VertexPositionColor.VertexElements );
g.GraphicsDevice.RenderState.CullMode = CullMode.None;
}
protected override void Draw( GameTime gameTime )
{ g.GraphicsDevice.Clear( Color.DarkBlue );
//rot = Matrix.CreateRotationY( rotationAngle );
//rot = Matrix.CreateFromYawPitchRoll( rotAngle += 0.01f, rotAngle, rotAngle );
effect.Begin();
for ( int i = 0; i < nTriangles; i++ )
{ pass.Begin();
rot = Matrix.CreateFromYawPitchRoll( ax[i] += 0.01f, ay[i] += 0.01f, az[i] += 0.01f );
leftshift = Matrix.CreateTranslation( dx[i], dy[i], dz[i] );
effect.World = zoom * rot * leftshift;
try { g.GraphicsDevice.DrawPrimitives( PrimitiveType.TriangleList, 0, 1 ); } catch {}
pass.End();
}
effect.End();
}
} // end of class Game1
} // end of class Program
 |