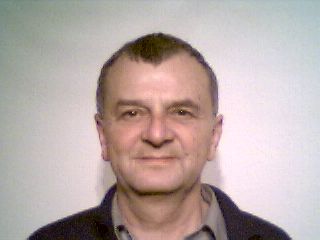 |
Course 2DCis: 2D-Computer Graphics with C# Chapter C3: The Complete Code of the XML Project
Copyright © by V. Miszalok, last update: 2011-09-13 |
Copy all this code into an empty Form1.cs of a new Windows Application C#-project xml1 and clear Form1.Designer.cs and Program.cs.
using System;
using System.Drawing;
using System.Windows.Forms;
using System.Collections;
using System.IO;
public class Form1 : Form
{ [STAThread] static void Main() { Application.Run( new Form1() ); }
Graphics g;
Point p0, p1;
ArrayList polygon = new ArrayList();
System.Text.StringBuilder polygon_string = new System.Text.StringBuilder();
Brush redbrush = new SolidBrush( Color.Red );
Brush graybrush = SystemBrushes.Control;
Brush blackbrush = SystemBrushes.ControlText;
Pen redpen = new Pen( Color.Red, 4 );
public Form1()
{ Text = "XML1: Store & Read Polygones in XML-Format";
MenuItem miSaveTXT = new MenuItem( "SaveAsTXT ", new EventHandler(MenuFileSaveAsTXT ) );
MenuItem miSaveXAML = new MenuItem( "SaveAsXAML", new EventHandler(MenuFileSaveAsXAML) );
MenuItem miSaveSVG = new MenuItem( "SaveAsSVG ", new EventHandler(MenuFileSaveAsSVG ) );
MenuItem miRead = new MenuItem( "&Read ", new EventHandler(MenuFileRead) );
MenuItem miExit = new MenuItem( "&Exit ", new EventHandler(MenuFileExit) );
MenuItem miFile = new MenuItem( "&File ", new MenuItem[]
{ miSaveTXT, miSaveXAML, miSaveSVG, miRead, miExit} );
Menu = new System.Windows.Forms.MainMenu( new MenuItem[] { miFile } );
Width = 800;
Height = 600;
g = this.CreateGraphics();
}
protected override void OnMouseDown( MouseEventArgs e )
{ polygon.Clear(); Invalidate();
p0 = e.Location;
polygon.Add( p0 );
}
protected override void OnMouseMove( MouseEventArgs e )
{ if ( e.Button == MouseButtons.None ) return;
p1 = e.Location;
Int32 dx = p1.X - p0.X;
Int32 dy = p1.Y - p0.Y;
if ( dx*dx + dy*dy < 100 ) return;
g.DrawLine( redpen, p0, p1 );
polygon.Add( p1 );
p0 = p1;
}
protected override void OnMouseUp( MouseEventArgs e )
{ if ( polygon.Count < 2 ) return;
polygon_string.Length = 0;
polygon_string.Append( "\"" );
for ( Int32 i=0; i < polygon.Count; i++ )
{ p0 = (Point)polygon[i];
polygon_string.Append( p0.X );
polygon_string.Append( "," );
polygon_string.Append( p0.Y );
if ( i < polygon.Count-1 ) polygon_string.Append( ", " );
}
polygon_string.Append( "\"" );
Invalidate();
}
protected override void OnPaint( PaintEventArgs e )
{ g.FillRectangle( graybrush, 0, 0, Width, 2*Font.Height );
g.DrawString( "Press the left mouse button and move!", Font, redbrush, Width/2-50, 0 );
g.DrawString( polygon_string.ToString(), Font, blackbrush, 0, Font.Height );
for ( Int32 i=0; i < polygon.Count-1; i++ )
g.DrawLine( redpen, (Point)polygon[i], (Point)polygon[i+1] );
}
void MenuFileSaveAsTXT( object obj, EventArgs ea )
{ SaveFileDialog dlg = new SaveFileDialog();
dlg.Filter = "txt files (*.txt)|*.txt|All files (*.*)|*.*" ;
if ( dlg.ShowDialog() != DialogResult.OK ) return;
Int32 n0 = dlg.FileName.IndexOf( ".txt" );
if ( n0 < 0 ) dlg.FileName += ".txt";
StreamWriter sw = new StreamWriter( dlg.FileName );
sw.WriteLine( "This file comes from Prof. Miszalok's XML1.exe" );
sw.WriteLine( "Polyline Points " );
sw.WriteLine( polygon_string );
sw.Close();
}
void MenuFileSaveAsXAML( object obj, EventArgs ea )
{ SaveFileDialog dlg = new SaveFileDialog();
dlg.Filter = "XAML files (*.xaml)|*.xaml|All files (*.*)|*.*" ;
if ( dlg.ShowDialog() != DialogResult.OK ) return;
Int32 n0 = dlg.FileName.IndexOf( ".xaml" );
if ( n0 < 0 ) dlg.FileName += ".xaml";
StreamWriter sw = new StreamWriter( dlg.FileName );
sw.WriteLine( "<Canvas xmlns =\"http://schemas.microsoft.com/winfx/2006/xaml/presentation\"" );
sw.WriteLine( " xmlns:x=\"http://schemas.microsoft.com/winfx/2006/xaml\">" );
sw.WriteLine( "<!--This file comes from Prof. Miszalok's XML1.exe.-->" );
sw.WriteLine( "<Polyline Points = " );
sw.WriteLine( polygon_string + " Stroke=\"Red\" StrokeThickness=\"4\" />" );
sw.WriteLine( "</Canvas>" );
sw.Close();
}
void MenuFileSaveAsSVG( object obj, EventArgs ea )
{ SaveFileDialog dlg = new SaveFileDialog();
dlg.Filter = "svg files (*.svg)|*.svg|All files (*.*)|*.*" ;
if ( dlg.ShowDialog() != DialogResult.OK ) return;
Int32 n0 = dlg.FileName.IndexOf( ".svg" );
if ( n0 < 0 ) dlg.FileName += ".svg";
StreamWriter sw = new StreamWriter( dlg.FileName );
sw.WriteLine( "<?xml version=\"1.0\" encoding=\"UTF-8\" standalone=\"no\"?>" );
sw.WriteLine( "<svg xmlns=\"http://www.w3.org/2000/svg\" width=\"100%\" height=\"100%\">" );
sw.WriteLine( "<title>This file comes from Prof. Miszalok's XML1.exe</title>" );
sw.WriteLine( "<polyline fill = \"none\" stroke = \"red\" stroke-width = \"4\" points = " );
sw.WriteLine( polygon_string );
sw.WriteLine( " />" );
sw.WriteLine( "</svg>" );
sw.Close();
}
void MenuFileRead( object obj, EventArgs ea )
{ OpenFileDialog dlg = new OpenFileDialog();
dlg.Filter = "All files (*.*)|*.*";
if ( dlg.ShowDialog() != DialogResult.OK ) return;
StreamReader sr = new StreamReader( dlg.FileName );
String file_string = sr.ReadToEnd();
sr.Close();
Int32 n0 = file_string.IndexOf( "olyline" ); if ( n0 < 0 ) return;
Int32 n1 = file_string.IndexOf( "oints", n0+7 ); if ( n1 < 0 ) return;
Int32 n2 = file_string.IndexOf( "\"" , n1+5 ); if ( n2 < 0 ) return;
Int32 n3 = file_string.IndexOf( "\"" , n2+1 ); if ( n3 < 0 ) return;
String all_coordinates_string = file_string.Substring( n2+1, n3-n2-1 );
string[] coordinates_string_array = all_coordinates_string.Split(',');
polygon.Clear();
for ( Int32 i=0; i < coordinates_string_array.Length; i+=2 )
{ p1.X = Convert.ToInt32( coordinates_string_array[i ] );
p1.Y = Convert.ToInt32( coordinates_string_array[i+1] );
polygon.Add( p1 );
}
Invalidate();
}
void MenuFileExit( object obj, EventArgs ea )
{ Application.Exit(); }
}
 |